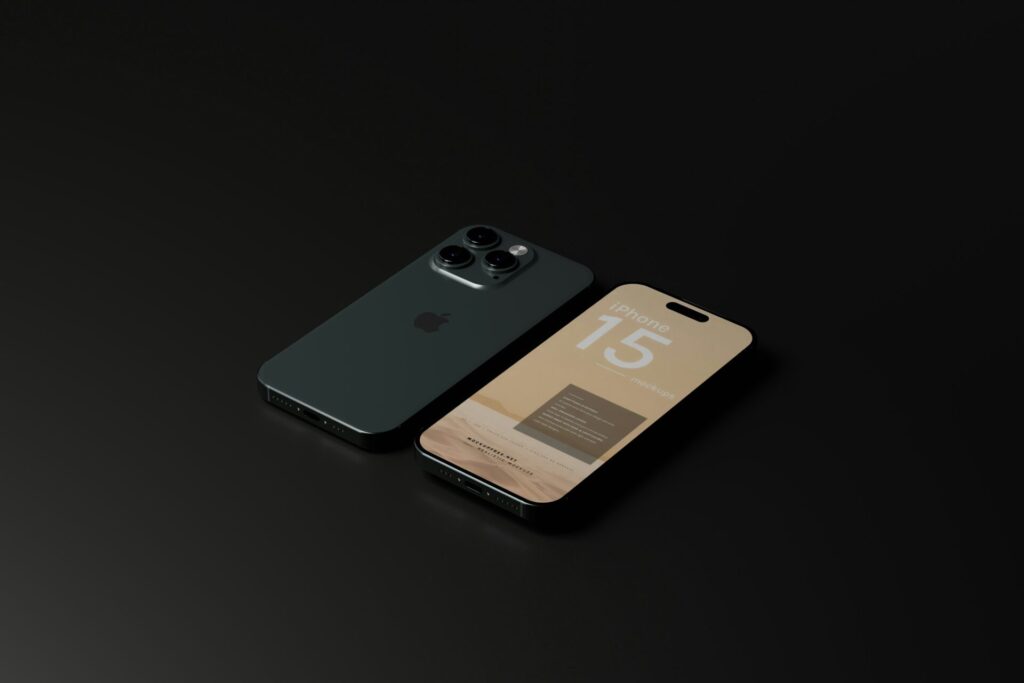
Every year, Apple releases a new iPhone, gradually increasing the size of RAM and main memory, adding power to the chip. Today, on iPhone 15, you can already run console games like “Resident Evil 4.” And a logical question may arise – should I optimize the size of my application or can I not spend more time on it?
In short, it is still worth optimizing the size. In this article, I’ve gathered reasons why it’s essential to do so and provided some useful optimization methods.
Problems and Definitions
So, let’s get started with the most banal answer to the question “Why does size matter?” – The limitations of the App Store. App Store Connect simply won’t allow you to download a file that exceeds the specified size limit.
For iOS and tvOS apps, verify that your app doesn’t exceed the maximum file sizes on the supported operating systems. Your app’s total uncompressed size must be less than 4GB.
Apple Watch apps must be less than 75MB. In addition, each Mach-O executable file — for example, app_name.app/app_name — must not exceed these maximum file sizes. Link
The specific files they are referring to might be a bit confusing. To better understand this, let’s walk through the process of submitting your application to the App Store Connect.
.xcarchive
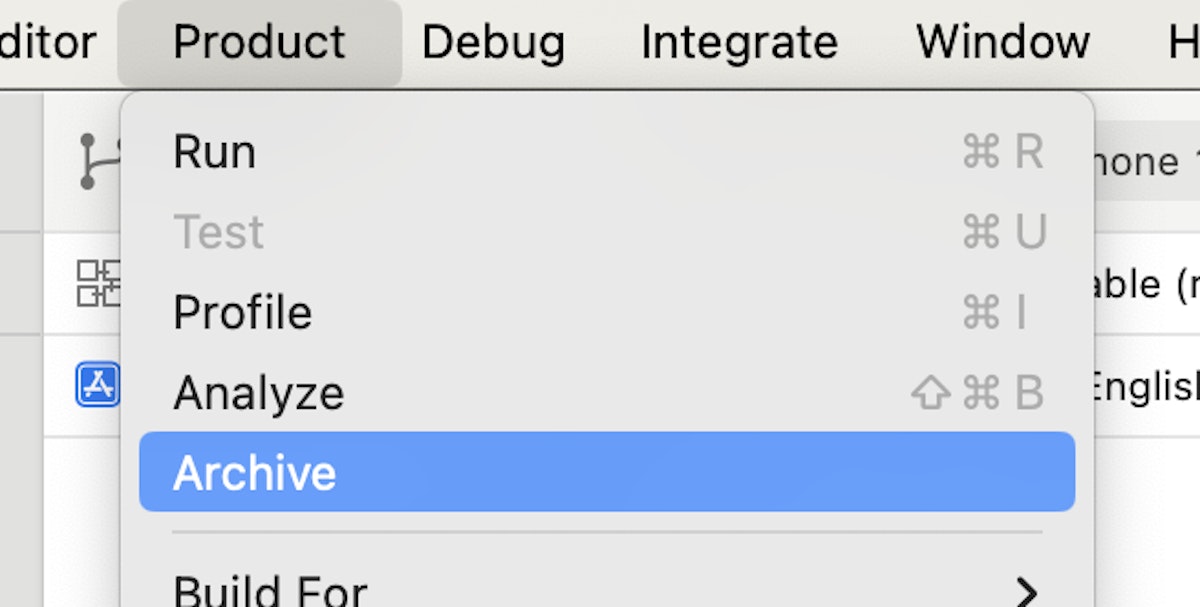
Product -> Archive
The first step is to create an archive. This archive stores a collection of build artifacts and related information for an iOS, macOS, watchOS, or tvOS app.
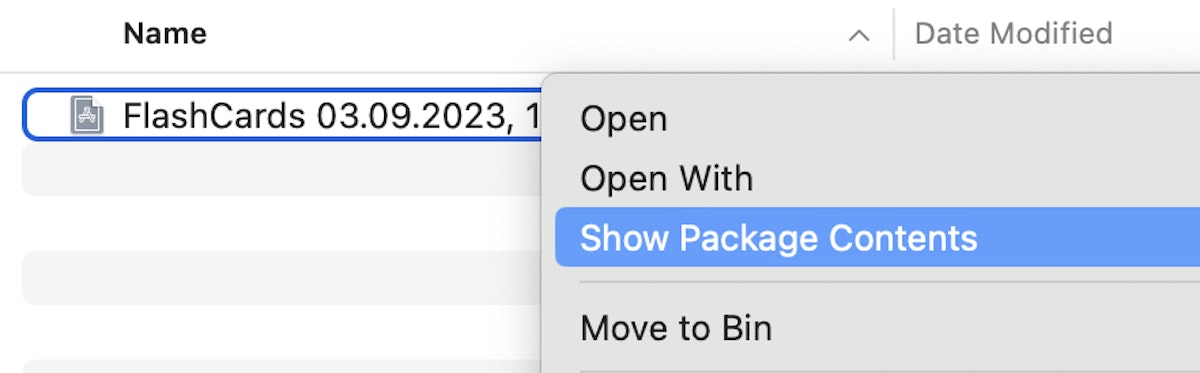
.xcarchive right click -> Show Package Contents
We do have an opportunity to look into what exactly and in what form is included in the archive.
Among the main files, you will find:
- Products folder with your App;
- dSYMs, (short for “debugging symbols”), special files generated by Xcode with the information necessary for debugging, in short, crash logs;
- Info.plist;
By the way, the Application file can also be opened by “Show package contents”, and among the files, you will find executable and CodeResources, a result of code signing; it keeps track of the digital signatures of various application resources (images, etc.).
.ipa
Returning back to Xcode, after generating the Archive, the Distribute App
button is available to you. At this stage, .xcarchive turns into .ipa.
An .ipa file can be thought of as a compressed package that includes a “Payload” folder. Inside this “Payload” folder, is the essential “YourApp.app” bundle. Within the “.app” bundle, you’ll find all the critical components of your application, including resources like
- images;
- plist files;
- compressed nib files;
- the executable file;
Additionally, it houses code-signing resources to ensure the app’s integrity and security.
To look at the insides of your .ipa, click Export
after distribution, convert the type from .ipa to .zip, and just extract.
In summary, the .ipa file is the packaged application that end-users install on their iOS devices, while the .xcarchive is a developer-focused archive that contains various assets and build information for the application.
The .ipa is used for distribution, while the .xcarchive is used for debugging, archiving, and further development purposes. The executable, on the other hand, is the central code that performs the app’s functions and is contained within the .ipa package.
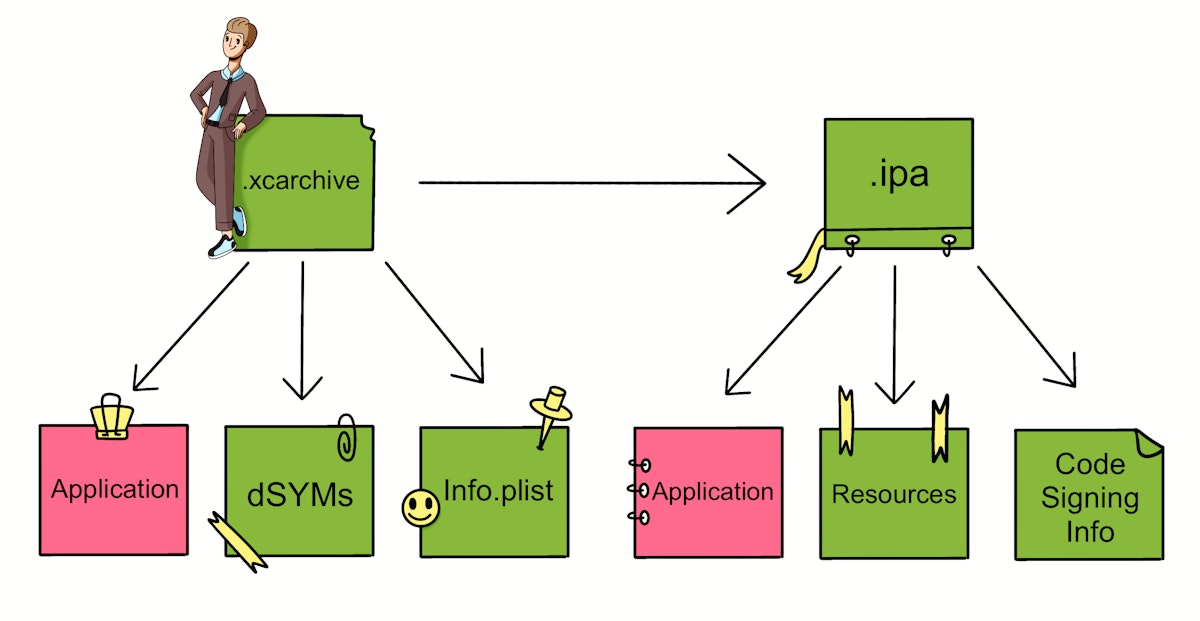
Thus, the limitations of the AppStore can be illustrated as follows.
OS version | .ipa size | .ipa -> Payload -> App -> exe size |
---|---|---|
iOS 9.0 and latertvOS 9.0 and later | 4 GB | 500 MB |
iOS 7.X through iOS 8.X | 2 GB | 60 MB |
However, to estimate the size of your final application, that is, how many bytes a particular user will have to install on their device, you will need additional actions, namely, generating an app size report. The documentation describes the procedure for creating it well, so I’ll leave a link here.
The next reason to think about the size of your application is … the AppStore again, but now, we are not talking about system restrictions but about the download speed. Everything is evident here – the smaller the size, the higher the rate.
Moreover, there is a limit of 200 MB, reaching which users need to connect to a Wi-Fi network to install the app. The delay can discourage users and lead to higher abandonment rates.
Apple’s App Store search and discovery algorithms often favor smaller apps, as they are easier for users to download and try out. Smaller app sizes can potentially improve your app’s visibility in search results and recommendations.
Once the app is on the device, its size still matters. Smaller apps launch faster, providing a better user experience. When an app optimizes storage, it contributes to extended battery life, app footprint reduction, and good device health. As a result, the more people are happy with the iPhone, the more potential users you have.
Solutions
There are some simple tips to avoid unnecessarily increasing the size of your application during development. The first of them is conscious work with images.
Images
First, choose HEIC instead of JPEG. HEIC provides files that are 50 percent smaller compared to JPEG, all while maintaining similar image quality. This results in reduced storage space on your device. Smaller files are easier to transfer files across networks, as well as quicker loading and saving to disk.
HEICs support image transparency and the capability to store supplementary images containing depth and disparity information. It supports lossless compression and enables you to store multiple images within a single container.
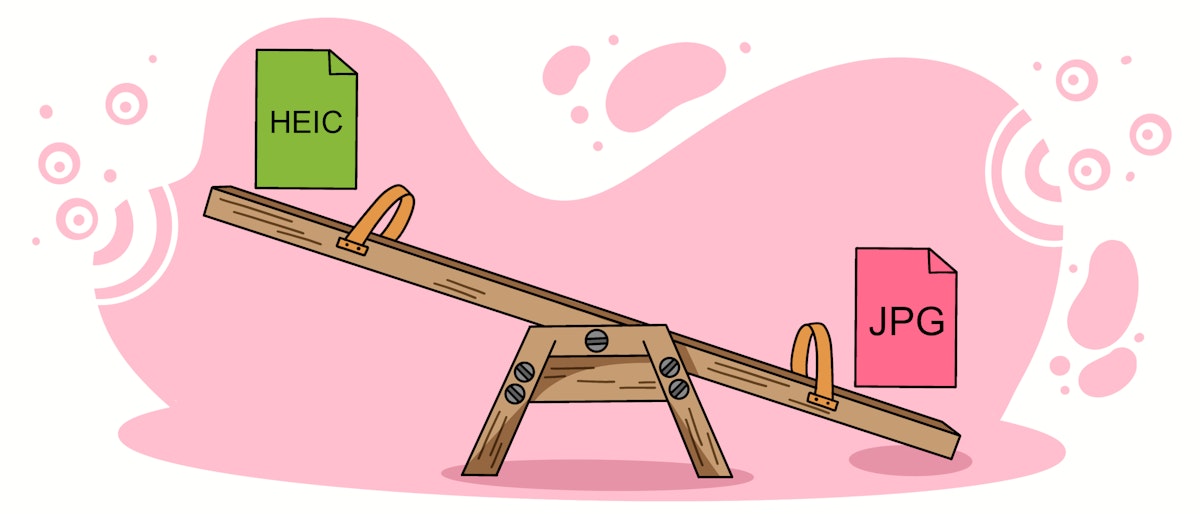
Secondly, try to adopt SVG (an XML-based vector image format used to display two-dimensional vector graphics) instead of PDF and PNG. In contrast to raster images, vector graphics typically exhibit smaller file sizes, because it is characterized by mathematical equations that define shapes and curves, as opposed to storing individual pixels.
Initially, it was necessary to add 3 images with prefixes (for each pixel density). Then PNG support was added (= vector image with a given size), but it still worked at the level of “cut 3 PNGs from PDF when we assemble the project.”
And only then did it become possible to use SVG + include the “use vector date” checkbox in the asset catalog, thereby really reducing the size of the images used + adding the possibility of infinite scaling, without loss of quality.
Thirdly, make the most of the capabilities ofAsset Catalogs. Asset Catalogs provide an easy-to-use storage for multiple resolutions of the same image. Moreover, catalogs store all image assets in a single optimized format with metadata instead of individual files.
It allows the App Store to provide only the necessary assets for their particular device. This leads to increasing download speed, and we already know that users do not like to wait.
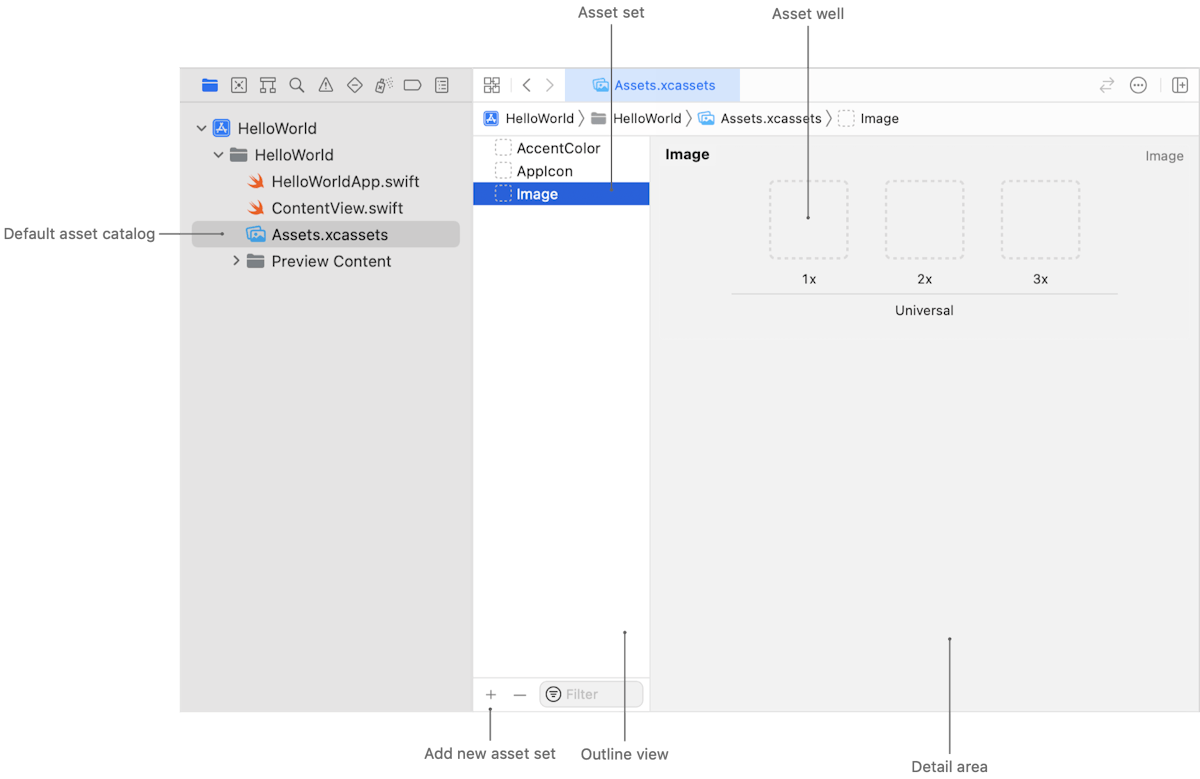
Asset Catalogs
It is possible to set “on-demand” on the resource, that is, the resource will be downloaded on the device only if necessary, and after some time of disuse, it will be removed. Link
Do not forget that you have a huge catalog of “free” images – SF Symbols. Apple is constantly working on increasing the characters, adding the ability to customize colors and even animations.
So, with pictures and other graphic resources, everything seems to be clear – we use the correct formats, and add a catalog through the Assets. There is always an opportunity not to include large resources in the final assembly, but simply upload from the Internet when needed. Now, let’s talk about the code and the use of libraries.
Frameworks Management
Let me quickly remind you about Linking. There are two types of it: static and dynamic.
Static | Dynamic | |
---|---|---|
When linking occurs | Build time | Runtime |
Where dependencies are stored | In the final executable file | In separate dynamic libraries |
How dependencies are shared | The same copy is used by all instances of the app | Each instance of the app has its own copy |
How updates to dependencies are handled | Rebuild the app | Update the dynamic library |
According to the theme of this article, dependencies storage is especially important to us, and dynamic linking looks like our favorite.
Dynamic libraries are not statically linked into client apps; they don’t become part of the executable file. Instead, dynamic libraries can be loaded (and linked) into an app either when the app is launched or as it runs. Link
Long story short, opting for dynamic libraries over static libraries results in smaller app file sizes and lower initial memory usage. However, it’s still important to strike a balance and avoid excessive use of dynamic libraries, as this can create a performance delay during the app’s start.
Apple also recommends creating a modular code base (SPM) in your app, which can turn out to be handy when sharing code with other targets, App Clipps, for example.
Swift Package Manager offers a streamlined and native way to manage dependencies in your Swift projects.
Excess Files
One of the most effective ways to reduce your app size is removing all unnecessary files. These extra files can be, for example, Read.me or leftover images. In fact, at the very beginning of the article, where we figured out what an .ipa is, we already learned how to find all the files that will get into the AppStore: .ipa -> .zip -> App -> show package contents.
Find out all the resources that you do not need, and feel free to delete them from your app.
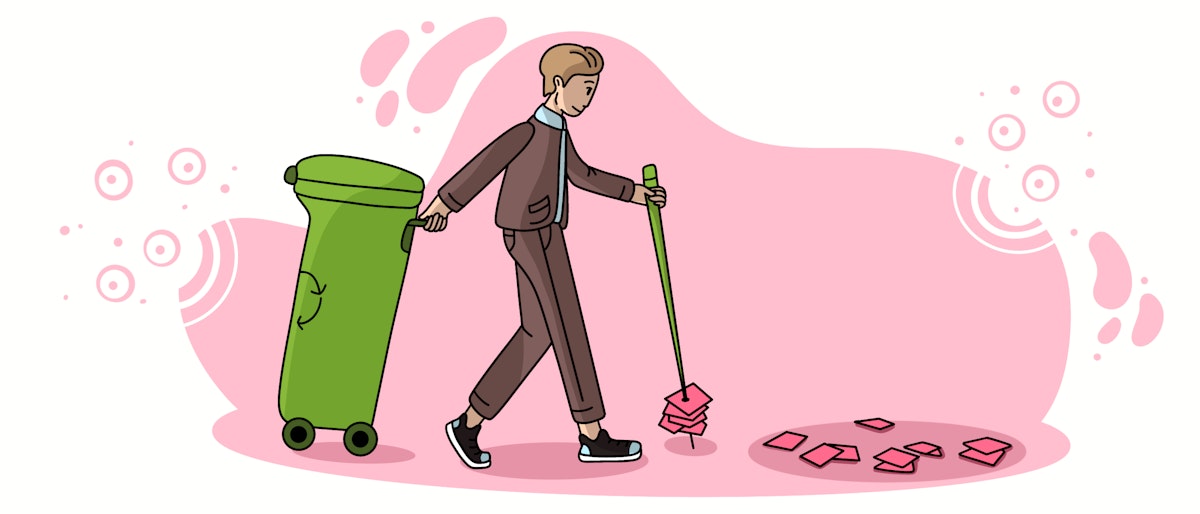
Conclusion
Just to bottom line this. There are still some important reasons why we should keep an eye on our app size:
- App Store limits;
- Download and launch speeds;
- Influence on device’s battery life;
And there are some methods to reduce app size:
- HEIC and SVG formats for images;
- Asset Catalogs;
- Dynamic linking;
- Filtering excess files;
So just don’t forget about it during your routine development; become smarter every day 🙃
This article was originally published by Daria Leonova on Hackernoon.